The E-Learning Project Review (3) Coding
At this stage, we started writing the system. The following tools were used for development.
Text Editor |
|
Browser |
|
Browser Extension | Winodw Resizer Beta on Chrome |
Local Web Server | MAMP |
FTP Client | Filezilla |
MAMP
MAMP is a tool available on Apple macOS and Microsoft Windows. It can easily deploy an Apache server on the localhost. My job was to desing the UI. Since we used PHP language to write web program, the .php file could not run directly on the localhost w/o a web server. To deploy a local web server allowed me to test and modify the UI on my computer and not always to upload newly modified code instantly to the Queen.
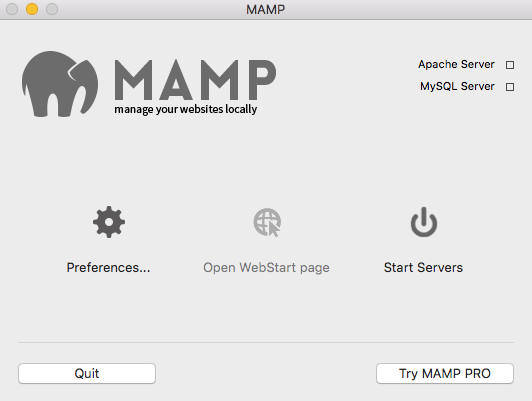
MAMP
Window Resizer
Since NLIS required us to add Responsive web design (RWD) feature to the system, I used this chrome extension to test the system UI and check if all the content were correctly displayed on different size of the screen.
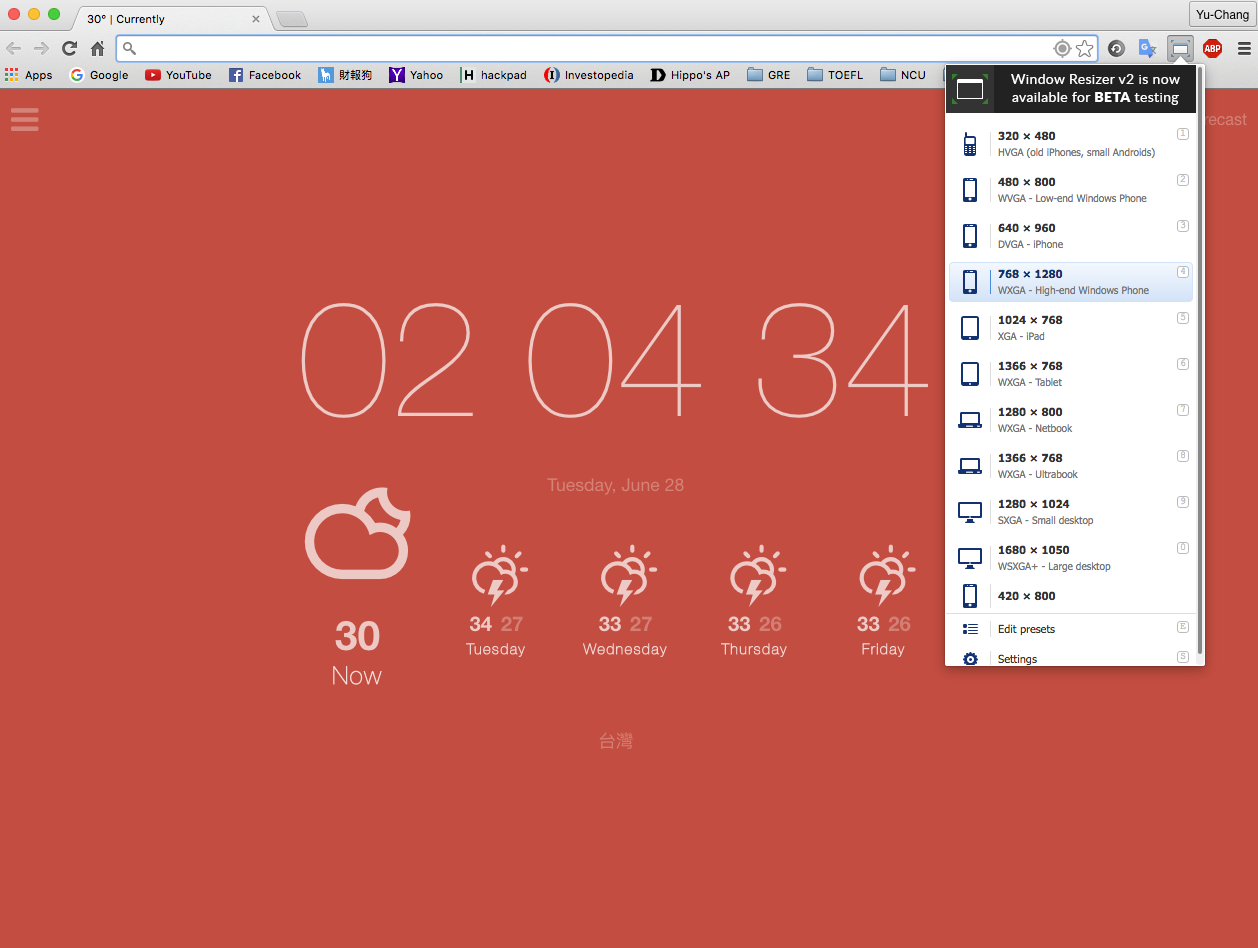
The Window Resizer Extension
The UI of Our System
Cascading Style Sheets (CSS) is a style sheet language used for describing the presentation of a document written in a markup language. - Wikipedia
There are 3 ways to enable CSS settins on the html components.
- Write it directly in side the web page file (.html, .php,.etc)
- Define in the HTML tag using
style=""
- Make a .css file and link it to the web page (using relative path)
Write it directly in side the web page file
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>CSS inside</title>
<!-- CSS code here! -->
<style>
.hippo
{
border: #ff0000 2px solid;
font-size: 30px;
background-color: #787878;
}
</style>
</head>
<body>
<div class="hippo">
Hello! This is class hippo.
</div>
</body>
</html>
Define in the HTML tag
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>CSS UsingClass</title>
</head>
<body>
<!-- Writing CSS code inside HTML Tag -->
<div style="border: #ff0000 2px solid; font-size: 30px; background-color: #787878;">
Hello! This is the 1st div.
</div>
<br />
<!-- Writing CSS code inside HTML Tag -->
<div style="border: #0000ff 2px solid; font-size: 40px; background-color: #d5d5d5;">
Hello! This is the 2nd div.
</div>
</body>
</html>
Link .css file to the web page
index.html
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>CSS outside</title>
<!-- CSS Outside! -->
<link rel="stylesheet" type="text/css" href="./test.css">
</head>
<body>
<div class="hippo">
Hello! This is class hippo.
</div>
</body>
</html>
test.css
.hippo
{
border: #ff0000 2px solid;
font-size: 30px;
background-color: #787878;
}
The UI of our system changed for 3 times. The first look is my practice of CSS coding w/o using third-party framework.
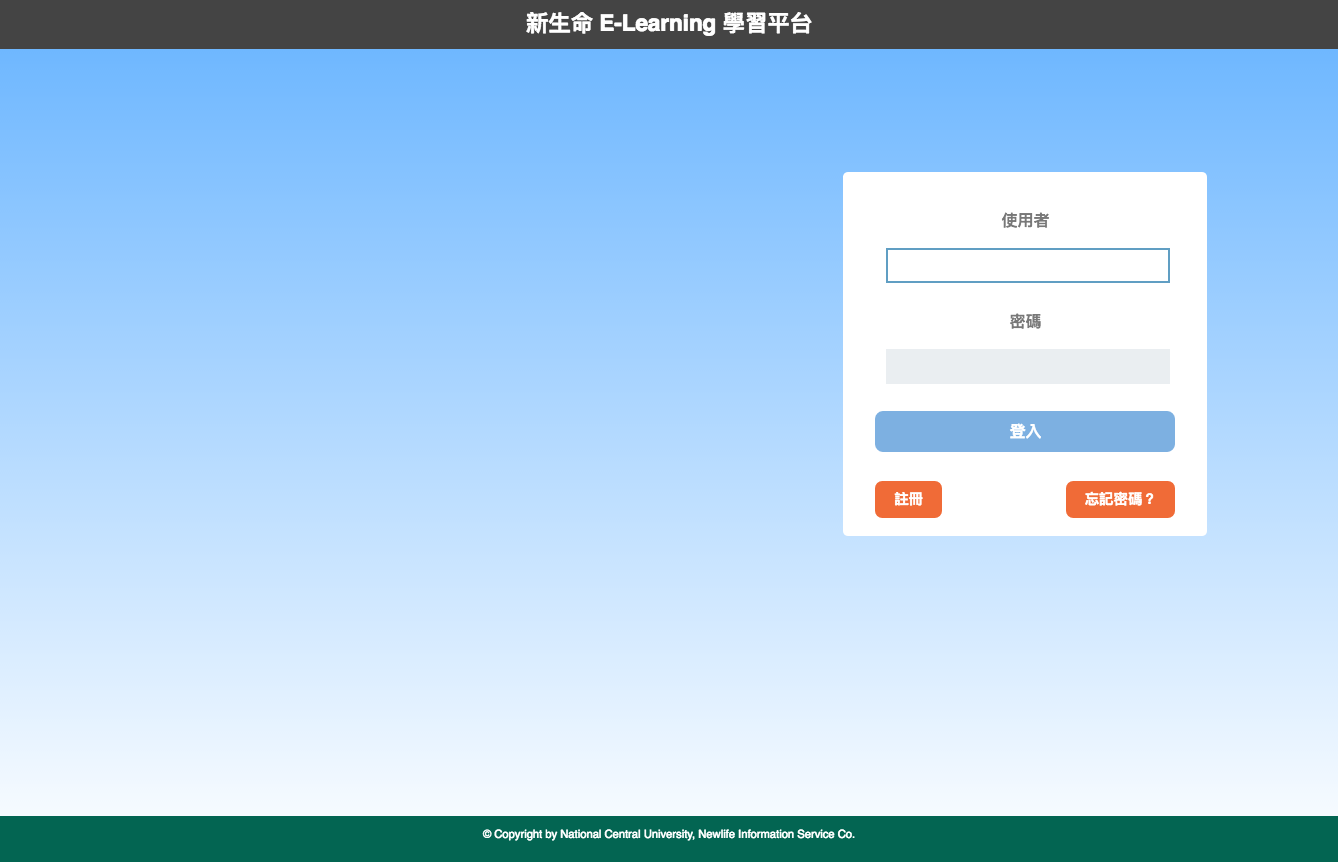
The 1st version of the UI
Since the second version, I started using Bootstrap framework which make the UI more convenient to design.
Bootstrap is a free and open-source front-end web framework for designing websites and web applications. It contains HTML- and CSS-based design templates for typography, forms, buttons, navigation and other interface components, as well as optional JavaScript extensions. - Wikipedia
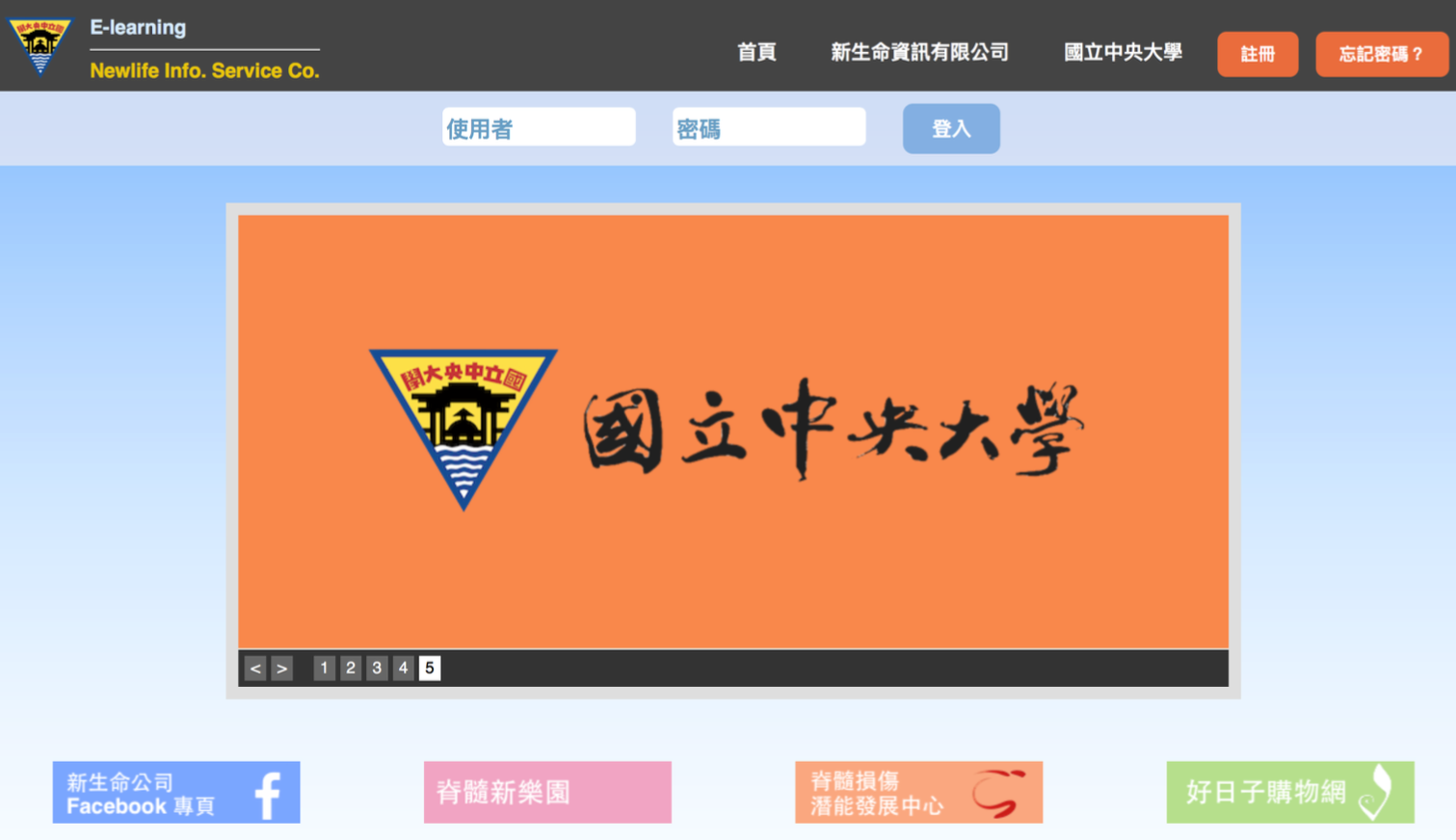
The 2nd version of the UI
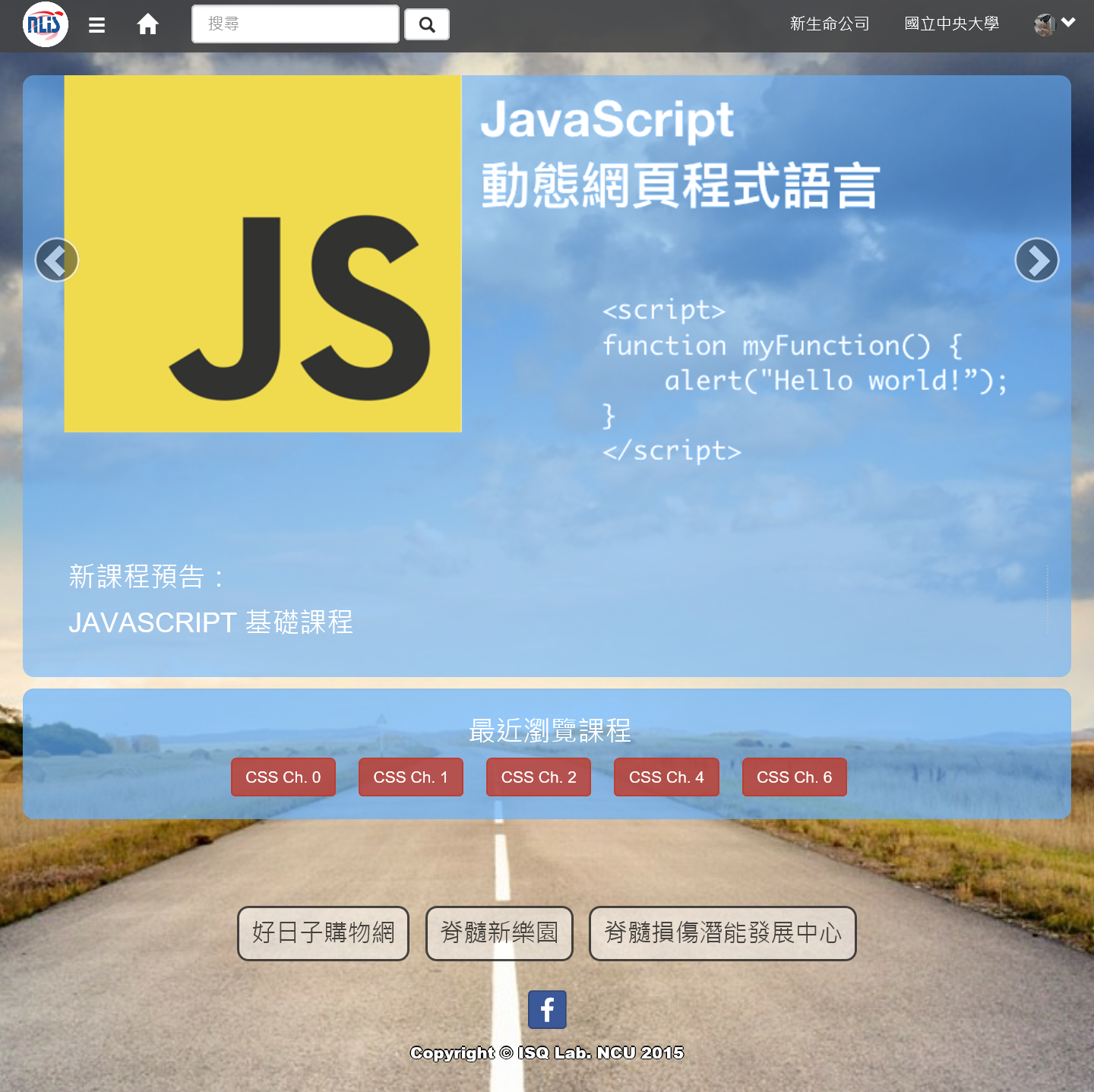
The 3rd version of the UI
Sample Code of RWD
@media (min-width: 768px)
{
.[class]
{
/* Do Something */
}
}
My understanding is that the RWD feature needs the CSS settings to be relative. For example, when we added an image on the page, we might set the width of it, like width: ???px;
. However, to make it able to dynamically resize as the screen size change, the setting should be change as width: ?%;
.
As the settings the sample code given, when the min-width
of the screen is 768px
, the browser will use the given settings for [class]
. Otherwide, the settings won’t be used.
Together with the relative CSS settings, the RWD feature is enabled.
The historical recod of the examination performance using Chart.js to draw the pie chart.
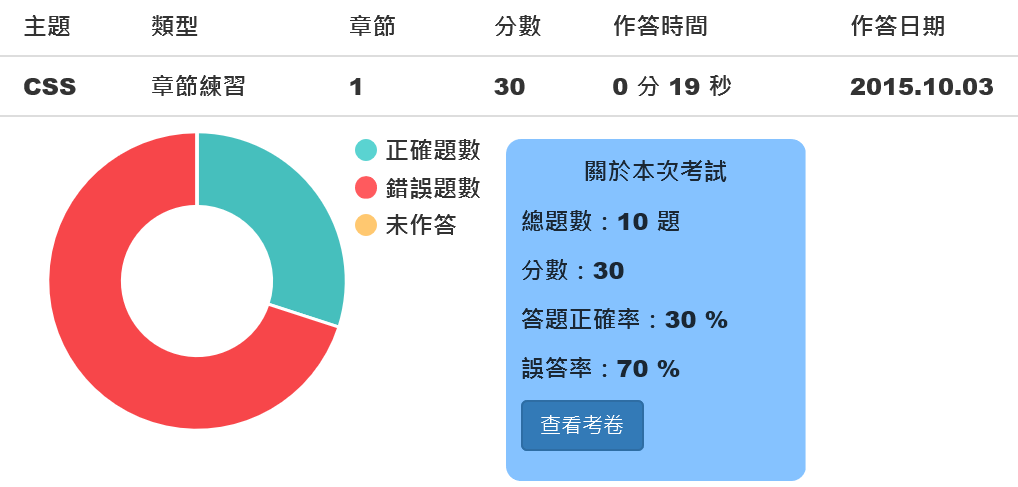
The Pie Chart using Chart.js
The Online Editor
To help the user practice while learning, I came up with an idea to embed an code editor on the class page. I found an sample code on the website, here’s the link.
The concept is to use javascript to load the code written in the <textarea>
to an <iframe>
.
First, get the document of the <iframe>
and the content inside the <textarea>
:
/* get the document of iframe: `<iframe name="dynamicframe"></iframe>` */
var d = window.dynamicframe.document;
/* get the content of textarea `<textarea id="htmlTA" spellcheck="false"></textarea>` */
var htmlText = window.editbox.document.getElementById("htmlTA").value;
or
$('#htmlTA').val();
Second, use an if
loop to check whether the content of <textarea>
has been modified. If so, update.
if( load != htmlText )
{
// update page content
load = htmlText;
d.open();
d.write(load);
d.close();
}
To allow the program could always get the newly modified code, it use window.setTimeout( [function name], 150);
to update cyclically.
Based on these concept, I modified the original version by added-on another <textarea>
to enable the user write customized CSS code.
Cosidering there might be some users want to restore their work online, I added-on php-download feature to allow them download their code.
User can download them partially. The 2 <textarea>
and the result shower all has a download button on it.
The one on the HTML code <textarea>
would download only the html page source written by the user online.
The second on the CSS code <textarea>
would download only the .css file.
The last one on the result shower would download the page source which combined the HTML code and CSS code written by the user online.
download.php
<?php
if(empty($_POST['filename']) || empty($_POST['content'])){
exit;
}
$filename = preg_replace('/[^a-z0-9\-\_\.]/i','',$_POST['filename']);
header("Cache-Control: ");
header("Content-type: text/plain");
header('Content-Disposition: attachment; filename="'.$filename.'"');
echo $_POST['content'];
?>
download.js
$(document).ready( function()
{
$('#download-btn').click( function(e)
{
var DC = $('#htmlTA').val();
$.generateFile({
filename : 'page.html',
content : DC,
script : './download.php'
});
e.preventDefault();
});
});
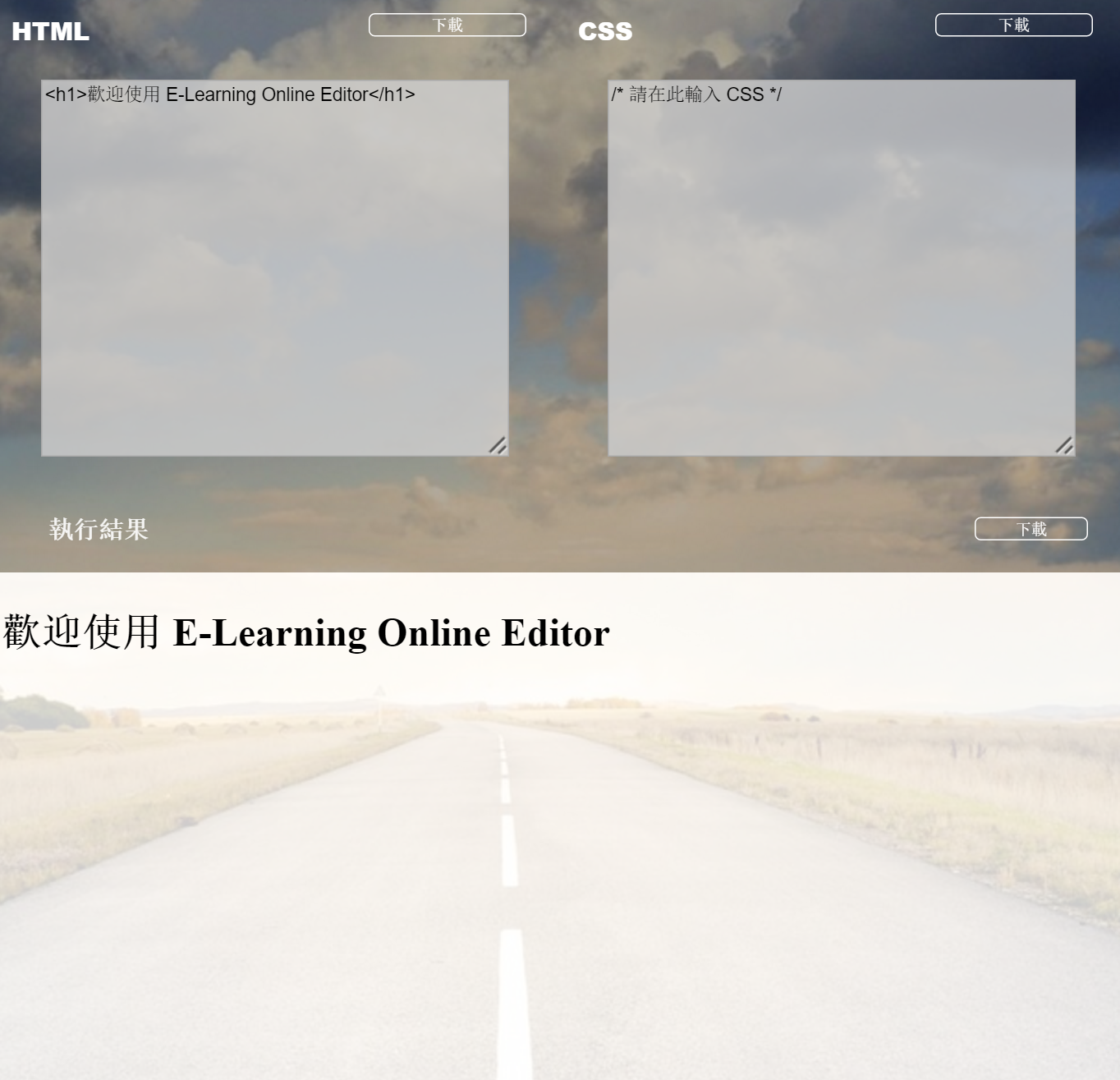
The Online Editor